time.sleep() in Python
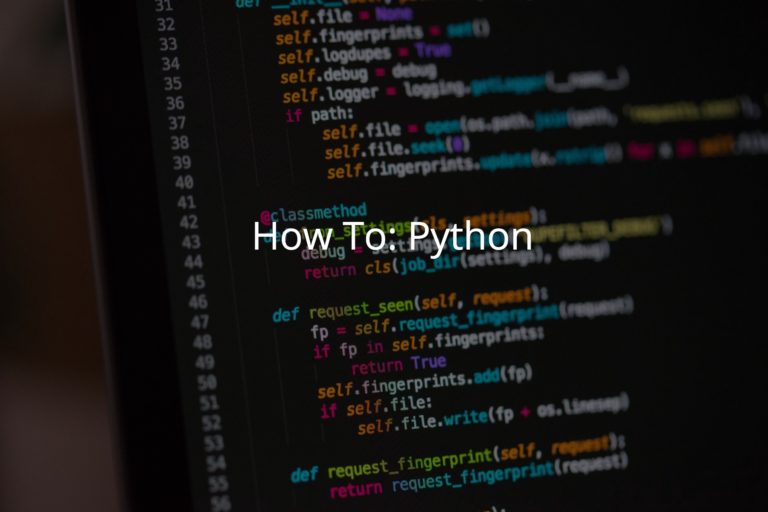
To make a Python program delay its execution for a given time, you can use the the time.sleep() method. The time module has a number of methods to deal with time and sleep() is one of them. The sleep() method…
To make a Python program delay its execution for a given time, you can use the the time.sleep() method. The time module has a number of methods to deal with time and sleep() is one of them. The sleep() method…
This is the 3rd article in a series on Object-Oriented Programming: Classes and Objects in Python Object-Oriented Programming: Encapsulation in Python Inheritance in Python Object-Oriented Programming: Polymorphism in Python Let’s define a generic Vehicle class and save it inside the…
This is the 1st article in a series on Object-Oriented Programming: Classes and Objects in Python Object-Oriented Programming: Encapsulation in Python Inheritance in Python Object-Oriented Programming: Polymorphism in Python Classes and Objects are the fundamental concepts of Object-Oriented Programming. In…
Errors are a part of every programmer’s life and knowing how to deal with them is a skill on its own. The way Python deals with errors is called ‘Exception Handling’. If some piece of code runs into an error,…
After some time the code starts to get more complex, with lots of functions and variables. To make it easier to organize the code we use Modules. A well-designed Module also has the advantage to be reusable, so you write…
As the code grows the complexity also grows, functions help organizing the code. Functions are a handy way to create blocks of code that you can reuse. Definition and Calling In Python use the def keyword to define a function.…
A list has its items ordered and you can add the same item as many times as you want. An important detail is that lists are mutable. Initialization Empty List people = [] List with initial values people = ['Bob',…
A tuple is similar to the list: ordered, allows repetition of items. There is just one difference: a tuple is immutable. Initialization Empty Tuple people = () Tuple with initial values people = ('Bob', 'Mary') Adding in a Tuple Tuples…
You are on the process of building a module with the basic math operations add, subtract, multiply, divide called basic_operations saved in the basic_operations.py file. To guarantee everything is fine, you make some tests. def add(a, b): return a +…
Sets don’t guarantee the order of the items and are not indexed. A key point when using sets: they don’t allow repetitions of an item. Initialization Empty Set people = set() Set with initial values people = {'Bob', 'Mary'} Adding…