Using the Github API in Python
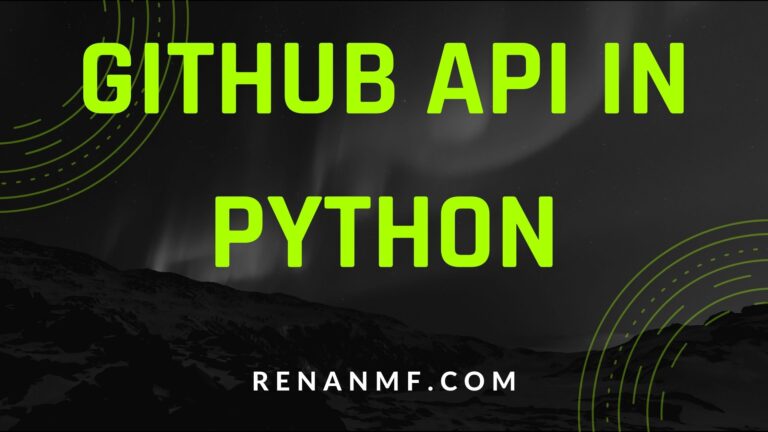
Github is a repository for developers to keep their projects and code versioned. You can create an account for free and use it as much as you want at no cost. Some APIs are paid and a bit troublesome to…
Github is a repository for developers to keep their projects and code versioned. You can create an account for free and use it as much as you want at no cost. Some APIs are paid and a bit troublesome to…
If you need and introduction to SQL and Databases, I recommend reading these articles before diving into this one: Introduction to SQL SQL: Tables and Basic Structure SQL: Data Types SQL: Syntax SQLite is a database that comes pre-installed with…
The IDLE (Integrated Development and Learning Environment) debugger is basically PDB with buttons and controls on a window, for those who prefer a more visual tool. The overall debugging process is: Set breakpoints Go through your code line-by-line checking variables…
Regular Expressions are also known simply as RegEx. Regular Expressions is one of those topics that scares most developers who don’t take their time to understand how they work properly. Having a strong notion of RegEx is like having an…
Linear Search is the most simple search algorithm. Considering you have a data structure, we have to go through each and every element of the data structure until we find the element we want. The implementation for a linear search…
With a worst case complexity of O(n^2), the Bubble Sort is the front door of the sorting algorithms. The algorithm loops through the array, comparing an item on the i position with the item on the i+1 position. In other…
String manipulation is one of those activities in programming that we, as programmers, do all the time. In many programming languages, you have to do a lot of the heavy lifting by yourself. In Python, on the other hand, you…
As of Python 3.10 we have a Python feature known as the Match-Case Statement. It was proposed on PEP 622 which was replaced by PEP 634 by the name of Structural Pattern Matching. match-case looks similar to the switch-case statement…
This is a confusion many people make. It is easy to look at lstrip() and removeprefix() and wonder what is the real difference between the two. When using lstrip(), the argument is a set of leading characters that will be…
Recursion happens when a function calls itself. The concept of a function calling itself is present in both math and programming. A recursive call prevents the use of while and for loops. Beware of Recursion Of course, as with any…