Functions in Python
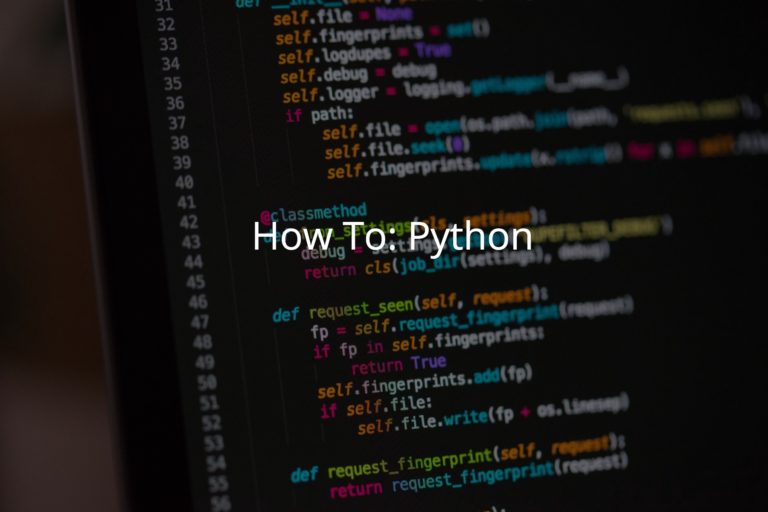
As the code grows the complexity also grows, functions help organizing the code. Functions are a handy way to create blocks of code that you can reuse. Definition and Calling In Python use the def keyword to define a function.…
As the code grows the complexity also grows, functions help organizing the code. Functions are a handy way to create blocks of code that you can reuse. Definition and Calling In Python use the def keyword to define a function.…
A list has its items ordered and you can add the same item as many times as you want. An important detail is that lists are mutable. Initialization Empty List people = [] List with initial values people = ['Bob',…
A tuple is similar to the list: ordered, allows repetition of items. There is just one difference: a tuple is immutable. Initialization Empty Tuple people = () Tuple with initial values people = ('Bob', 'Mary') Adding in a Tuple Tuples…
You are on the process of building a module with the basic math operations add, subtract, multiply, divide called basic_operations saved in the basic_operations.py file. To guarantee everything is fine, you make some tests. def add(a, b): return a +…
Sets don’t guarantee the order of the items and are not indexed. A key point when using sets: they don’t allow repetitions of an item. Initialization Empty Set people = set() Set with initial values people = {'Bob', 'Mary'} Adding…
You can run Python code directly on a terminal as commands or save the code in a file with .py extension and run the Python file. Terminal Running commands directly on a terminal is recommended when you want to run…
Python is known for its clean syntax. The language avoids using unnecessary characters to indicate some specificity. Semicolons Python makes no use of semicolons to finish lines. A new line is enough to tell the interpreter that a new command…
Python was created in 1990 by Guido Van Rossum in Holland. One of the objectives of the language was to be accessible to non-programmers. Python was also designed to be a second language to programmers due to its low learning…
If you use a Mac or Linux you already have Python installed, Windows doesn’t come with Python installed by default. But you might have Python 2 and we are going to use Python 3. Check if you have Python 3…
These operators provide an easy way to check if a certain object is present in a sequence: string, list, tuple, set, and dictionary. They are: in: returns True if the object is present not in: returns True if the object…