Type casting in Python
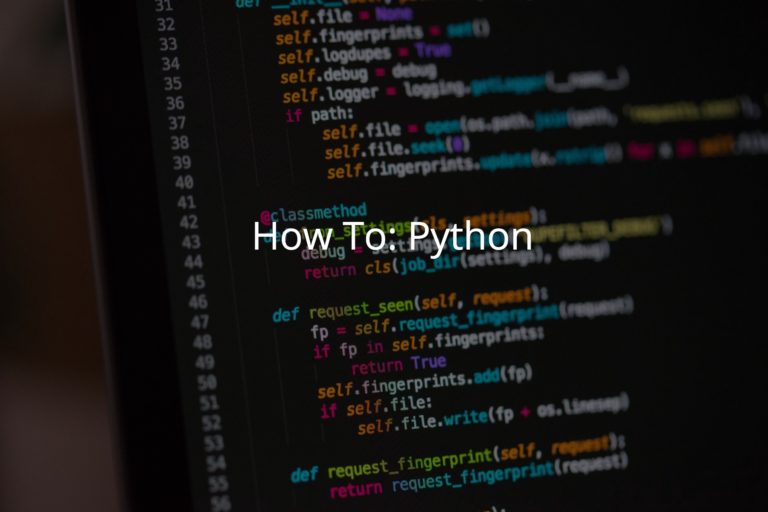
Explicit conversion To cast a variable to a string just use the str() function. my_str = str('32') # this is just a regular explicit intialization print(my_str) my_str = str(32) # int to str print(my_str) my_str = str(32.0) # float to…