isalpha(): checking letters only in a string in Python
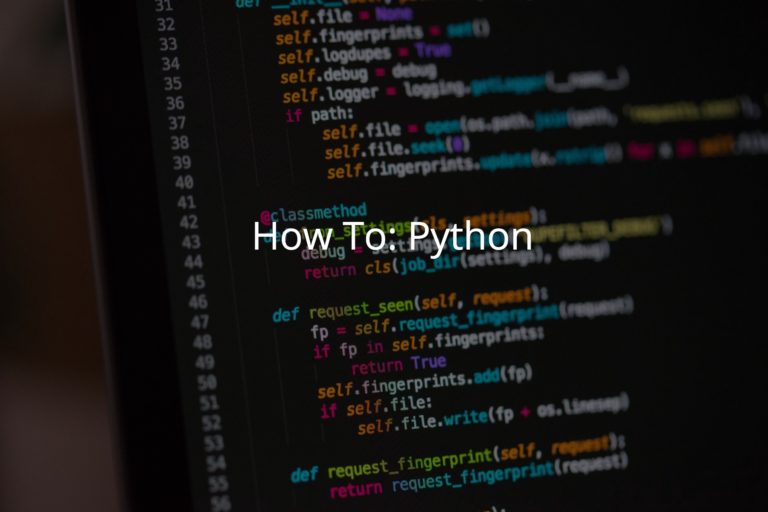
Use the isalpha() method to check if a string only contains letters. word = 'beach' print(word.isalpha()) #output: True word = '32' print(word.isalpha()) #output: False word = 'number32' print(word.isalpha()) #output: False word = 'Favorite number is blue' #notice the space between…